Building Scalable React Applications: Embracing Component-Based Architecture
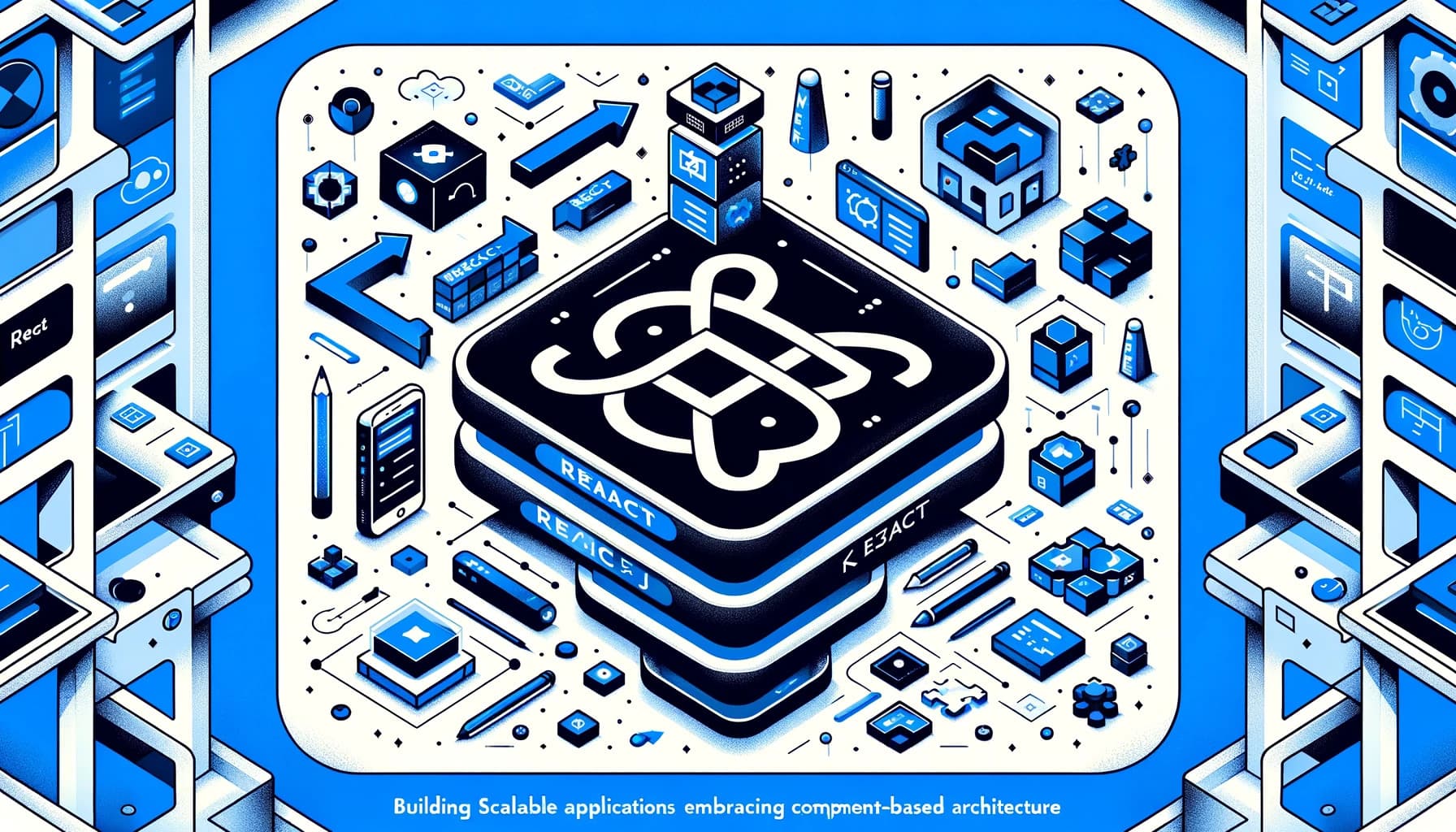
Until now, managing state in large-scale React applications has been a complex task that requires a deep understanding of component lifecycles, hooks, and Redux or Context API for global state management.
React's component-based architecture has revolutionized the way developers build user interfaces. By breaking down UIs into smaller, reusable components, React simplifies the development process and enhances the scalability of web applications. However, mastering state management within these components is crucial for building dynamic and responsive applications.
We often receive questions like:
How do I manage state effectively across multiple components? Should I use Redux, Context API, or hooks? Understanding when and how to utilize these state management strategies is key to developing efficient React applications. Each method has its advantages and is suited to different scenarios.
React Hooks, introduced in React 16.8, provide a more direct API to the React concepts you already know:
function CounterComponent() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
For more detailed explanations on managing state with hooks, consult the React documentation.
What to expect from here on out
Following this introduction, we'll explore various React concepts and best practices. This includes JSX syntax, functional vs. class components, React lifecycle methods, hooks, and optimizing application performance.
It's essential to cover all these topics comprehensively for several reasons:
- To ensure developers can build scalable and maintainable applications.
- To illustrate the power and flexibility of React's ecosystem.
- To provide insights into advanced React features and optimization techniques.
Let's delve deeper into React component patterns.
Embracing Component-Based Architecture
The beauty of React lies in its simplicity and the power of its component-based architecture.
Consider this when structuring your React applications:
Component composition is more powerful and flexible than inheritance. Favor composition over inheritance to reuse code between components.
React encourages the use of controlled components for input forms to manage form data:
class NameForm extends React.Component {
constructor(props) {
super(props);
this.state = {value: ''};
this.handleChange = this.handleChange.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
}
handleChange(event) {
this.setState({value: event.target.value});
}
handleSubmit(event) {
alert('A name was submitted: ' + this.state.value);
event.preventDefault();
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<label>
Name:
<input type="text" value={this.state.value} onChange={this.handleChange} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
}
Understanding and applying the concept of controlled components is crucial for handling form inputs effectively in React applications.
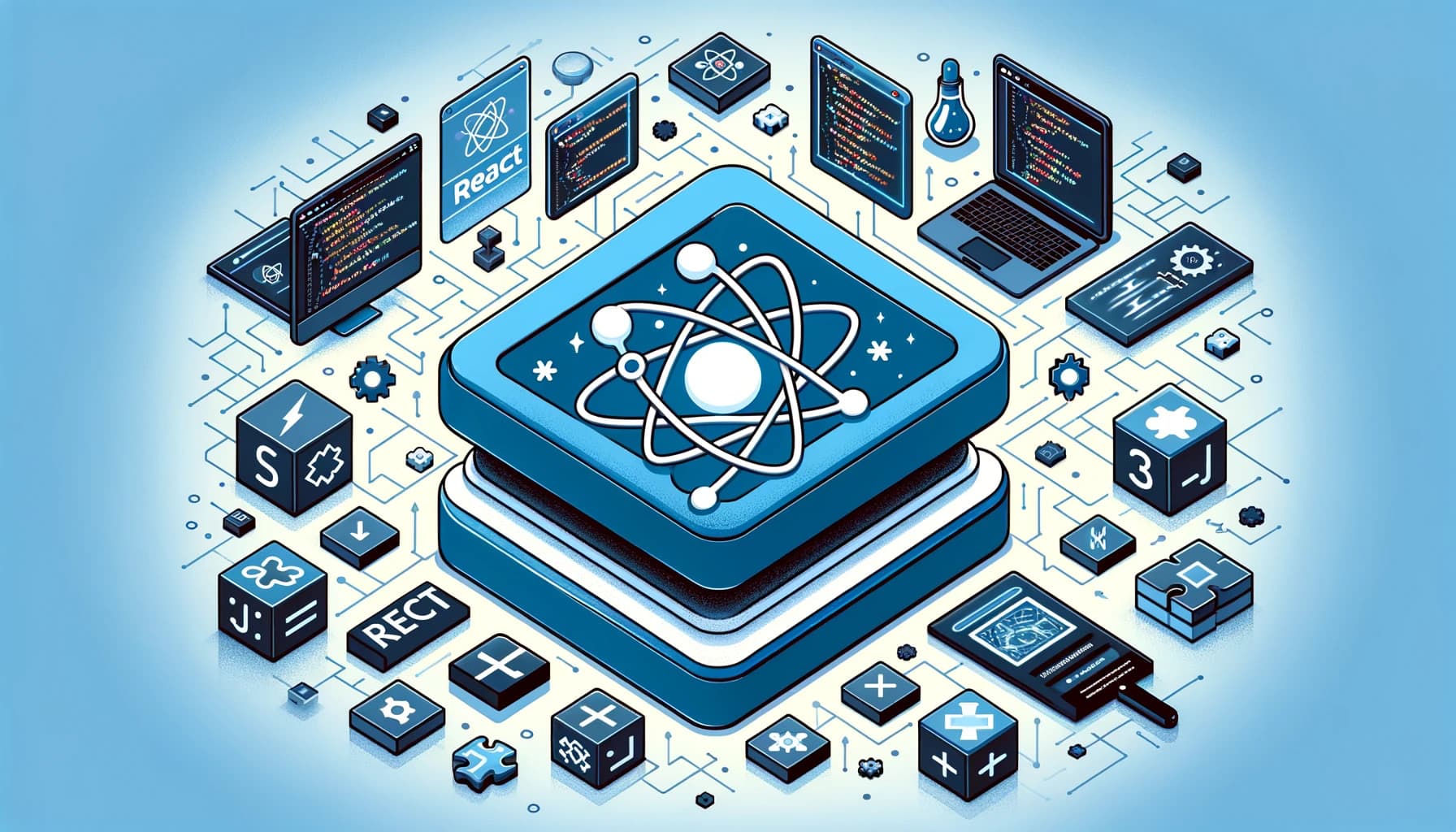
Handling Side Effects with useEffect
React's useEffect
hook allows you to perform side effects in function components. It's a cornerstone of interacting with APIs or executing any side effects:
useEffect(() => {
// Fetch data or perform side effects here
}, [dependency]);
This hook replaces several lifecycle methods in class components, providing a cleaner and more efficient way to handle side effects.
Code Splitting for Better Performance
To enhance your React application's performance, consider code splitting, which allows you to split your code into smaller chunks that are loaded only when needed:
const OtherComponent = React.lazy(() => import('./OtherComponent'));
Utilizing dynamic import()
syntax with React.lazy
for component rendering can significantly reduce the initial load time of your application.
Emphasizing Accessibility and Semantic HTML
Accessibility should be a priority in web development. React and JSX make it easy to integrate accessible elements into your components, ensuring your applications are usable by as many people as possible:
<button aria-label="Close" onClick={handleClose}>
Close
</button>
Incorporating ARIA attributes and semantic HTML elements within React components enhances accessibility and SEO, providing a better user experience.
Conclusion
Building scalable and maintainable React applications requires a solid understanding of component-based architecture, state management, and performance optimization techniques. By following React's best practices and leveraging its powerful features, developers can create efficient and robust web applications.
This article has covered the foundational concepts of React development, aiming to equip developers with the knowledge needed to tackle real-world challenges in React-based projects.